Image Tracking Sample
This sample demonstrates how to detect and augment image targets found in the real world.
For basic information about image tracking and what AR Foundation's AR Tracked Image Manager
component does, please refer to the Unity documentation.
In order to use this feature it has to be enabled in the OpenXR plugin settings located under Project Settings > XR Plug-in Management > OpenXR (> Android Tab)
.
How the sample works
First and foremost, make sure to have the Image Tracking
feature enabled in the OpenXR project settings.
Reference images can be found in the Image Targets for Testing section
Image targets are fed to the underlying XR plugin through an XR Reference Image Library
.
The added image has a name which will be useful for identifying a tracked target later on, and an important flag Keep Texture at Runtime which should set to true.
This enables the subsystem to pass the texture data to the OpenXR Runtime application for tracking purposes.
Additionally, the image target in the sample's case was measured at 26 cm in height (when printed in DIN A4 or US letter). The correct measures are essential for a correct pose estimation and subsequent placement of an augmentation. Therefore, it must be specified after enabling Specify Size.
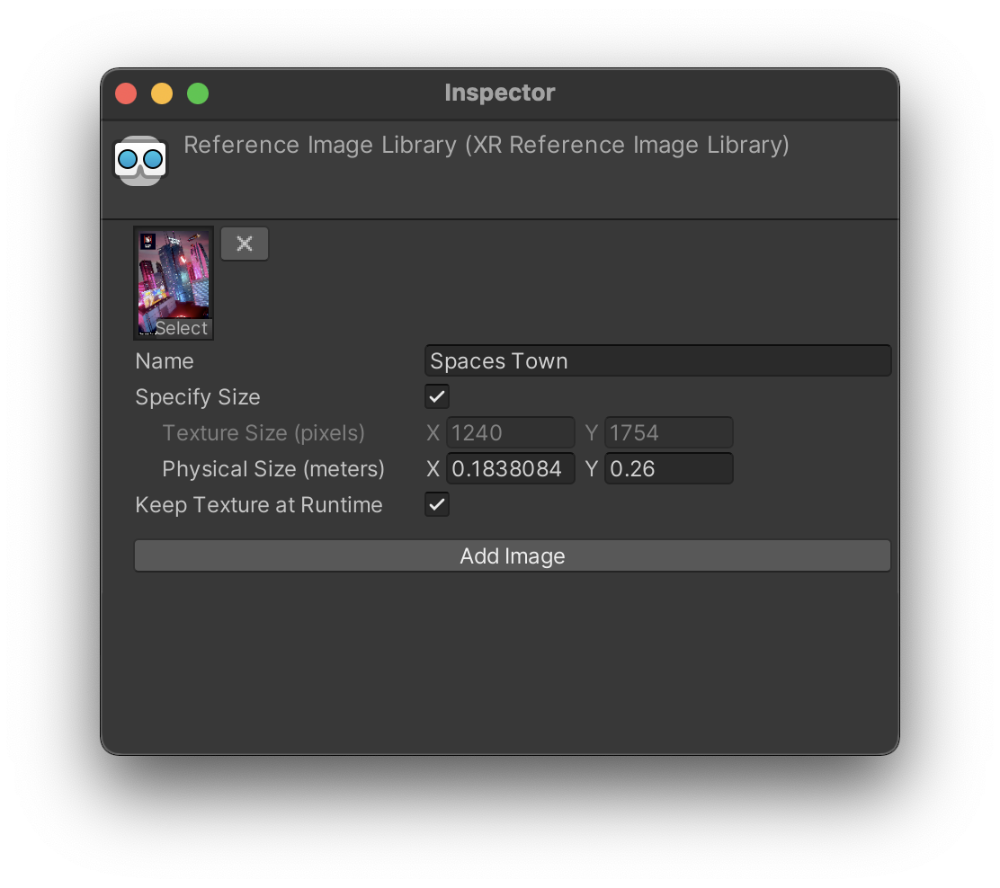
In the Import Settings the texture should have the Read/Write flag enabled and the Format set to RGB 24 bit (which may also appear as RGB8). If the Format is set to Automatic, the project's graphics settings may set the format incorrectly.
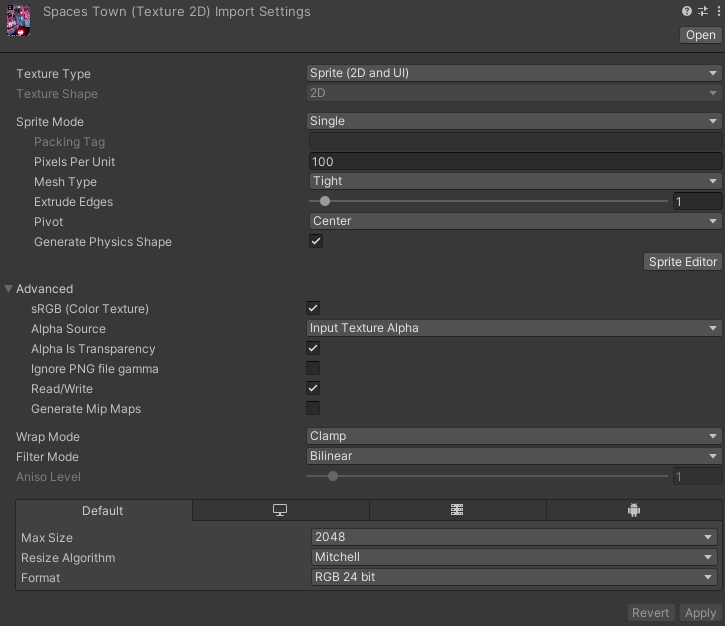
Adding the ARTrackedImageManager
to the XROrigin
GameObject will enable the Image Tracking Subsystem included in the Snapdragon Spaces package. The component provides three fields: a field for the RuntimeReferenceimageLibrary
created above, a field for specifying the Max Number Of Moving Images, and a field for defining a prefab to spawn upon detection of a tracked image. The prefab used is a gizmo that indicates the orientation of the tracked image.
By subscribing to the ARTrackedImageManager
's method to listen for tracked image changes, the appropriate UI information gets set for the tracking state and position of the tracked image, as seen in the simplified code example below.
For this sample to work the reference image names set in the XR Reference Image Library
need to be unique. Any identical names will cause a hash code collision in the _trackedImages
Dictionary.
...
private Dictionary<TrackableId, ...> _trackedImages = new Dictionary<TrackableId, ...>();
public override void OnEnable() {
...
FindObjectOfType<ARTrackedImageManager>().trackedImagesChanged += OnTrackedImagesChanged;
}
public override void OnDisable() {
...
FindObjectOfType<ARTrackedImageManager>().trackedImagesChanged -= OnTrackedImagesChanged;
}
private void OnTrackedImagesChanged(ARTrackedImagesChangedEventArgs args) {
foreach (var trackedImage in args.added) {
if (trackedImage.referenceImage.name == "Spaces Town") {
_trackedImages.Add(trackedImage.trackableId, ...);
...
}
}
foreach (var trackedImage in args.updated) {
var info = _trackedImages[trackedImage.trackableId];
...
}
foreach (var trackedImage in args.removed) {
_trackedImages.Remove(trackedImage.trackableId);
...
}
}