Local Anchors
How to Enable the Feature
In order to use the Local Anchors feature, it has to be enabled in the OpenXR plugin settings located under Project Settings > XR Plug-in Management > OpenXR (> Android Tab) > OpenXR Feature Groups > Snapdragon Spaces > Spatial Anchors.
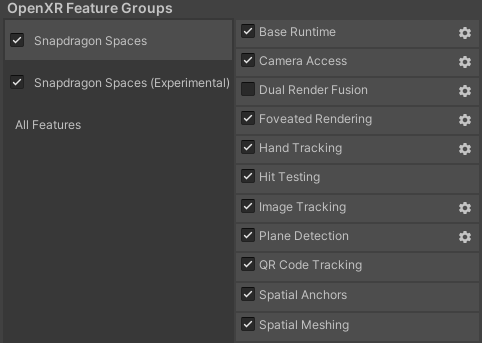
Spaces Anchor Store (Script)
Make sure to look around the environment in order to generate a better tracking map and reduce saving and loading times. Saving multiple anchors at once blocks the main thread, so the callback should be used to save any subsequent anchor.
This component requires an AR Anchor Manager component and will add one to the GameObject if none is already present. By adding a Spaces Anchor Store component next to the AR Anchor Manager, anchors can be saved locally to be recognized and tracked in a later session.
The component provides following APIs for loading and saving an anchor, deleting a saved anchor, and clearing the local storage of anchors.
namespace Qualcomm.Snapdragon.Spaces
{
public class SpacesAnchorStore
{
public void ClearStore();
public void SaveAnchor(ARAnchor anchor, string anchorName, Action<bool> onSavedCallback = null);
public void SaveAnchor(ARAnchor anchor, Action<bool> onSavedCallback = null);
public void SaveAnchorWithResult(ARAnchor anchor, string anchorName, Action<SaveAnchorResult> onSavedCallback = null);
public void SaveAnchorWithResult(ARAnchor anchor, Action<SaveAnchorResult> onSavedCallback = null);
public void DeleteSavedAnchor(string anchorName);
public void LoadSavedAnchor(string anchorName, Action<bool> onLoadedCallback = null);
public void LoadAllSavedAnchors(Action<bool> onLoadedCallback = null);
public string[] GetSavedAnchorNames();
public string GetSavedAnchorNameFromARAnchor(ARAnchor anchor);
}
}
Until this information moves to a scripting API, here is a short description of these methods:
ClearStore
- Clears the local storage of anchors.
SaveAnchor
- Saves an
AR Anchor
object either by a given name or by a generated hash. Can invoke a callback on complete.
- Saves an
SaveAnchorWithResult
- Saves an
AR Anchor
object either by a given name or by a generated hash. Can invoke a callback on complete. - Possible values for
SaveAnchorResult
are the following:PENDING
: Anchor is pending saving. Cannot be normally seen, therefore useARAnchor.pending
instead.SAVED
: Saved successfully in local storage.FAILURE_RUNTIME_ERROR
: Not saved in local storage due to a runtime error.FAILURE_STORE_NOT_LOADED
: Not saved in local storage due to Spaces Anchor Store failing to load.FAILURE_INSUFFICIENT_QUALITY
: Not saved in local storage due to insufficient quality of the environment map.
- Saves an
DeleteSavedAnchor
- Deletes a saved anchor by name from local storage.
LoadSavedAnchor
- Loads an anchor from the local storage and tries to locate the anchor in the scene. If the anchor was found, an
AR Anchor
object will be instantiated. Loaded anchors will be listed asadded
in ARAnchorManager'sanchorsChanged
event. The name of the saved anchors can be retrieved withGetSavedAnchorNames
. Can invoke a callback on complete.
- Loads an anchor from the local storage and tries to locate the anchor in the scene. If the anchor was found, an
LoadAllSavedAnchors
- Loads all anchors from storage and tries to locate them in the scene. Similar as with
LoadSavedAnchor
, anAR Anchor
object will be instantiated upon its recognition.
- Loads all anchors from storage and tries to locate them in the scene. Similar as with
GetSavedAnchorNames
- Returns all saved anchors by name.
GetSavedAnchorNameFromARAnchor
- If a tracked
AR Anchor
object is a formerly saved one, this method will return its name, otherwise an empty string. This method can be used to check if an anchor is a saved one or not.
- If a tracked