QR Code Tracking
How to Enable the Feature
In order to use QR Code Tracking, it has to be enabled in the OpenXR plugin settings located under Project Settings > XR Plug-in Management > OpenXR (> Android Tab) > OpenXR Feature Groups > Snapdragon Spaces > QR Code Tracking.
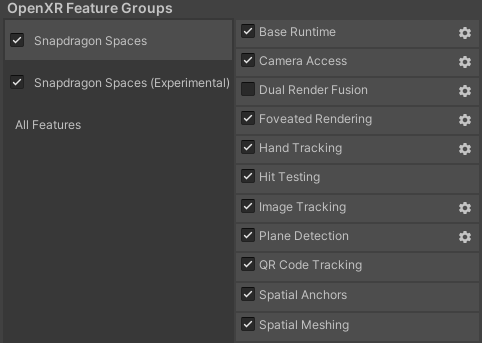
Spaces QR Code Manager (Script)
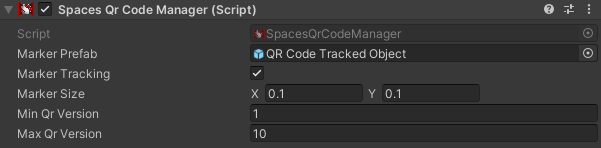
Adding the SpacesQrCodeManager component to the XR Origin GameObject will enable the QR code Tracking Subsystem included in the Snapdragon Spaces package. The component provides six (6) fields:
- The Marker Prefab object is instantiated every time a QR code is detected. Tracking will work only if Marker Tracking is enabled.
- The
Marker Tracking Mode
is set for all tracked markers. For more information on what eachTracking Mode
does, see the Image Tracking documentation.
Tracking Mode | Added | Updated | Removed |
---|---|---|---|
Dynamic (default) | Marker detected | Every frame | Marker detection lost |
Static | Marker detected | Never | New marker tracked |
Adaptive | Marker detected | Immediately with head movement and periodically for marker movement | Marker detection lost |
- The
Marker Tracking
field enables and disables the tracking capabilities of the feature. QR code detection will run continuously, but tracking can be disabled if not needed. Marker Size
is the width and height in meters of the physical markers to be detected. Correct measurements are essential for accurate pose estimation and subsequent placement of any augmentations.Minimum QR Version
andMaximum QR Version
are the versions of QR codes which will be detected. Any QR codes outside of this range will not be detected or tracked.
Subscribing to the SpacesQrCodeManager::OnMarkersChanged
method allows developers to use a familiar ARFoundation pattern to get the position, orientation, and string data of the tracked marker, as seen in the simplified code example below.
private override void Start() {
FindObjectOfType<SpacesQrCodeManager>().markersChanged += OnMarkersChanged;
}
private void OnMarkersChanged(SpacesMarkersChangedEventArgs args) {
foreach (var marker in args.added) {
...
}
foreach (var marker in args.updated) {
...
}
foreach (var marker in args.removed) {
...
}
}
- Maximum recognition distance decreases with higher QR code versions.
- To ensure recognition from a distance of 1 meter or more, it is recommended to use QR codes with a version number between 1 and 6.
- QR codes can be detected at a viewing angle of up to 45 degrees.
- There is limited support for artistic QR codes. Standard black on white rectangular module patterns are recognized most easily. Logo's are supported but GenAI QR codes are unlikely to work.
- Printing the QR code on non-reflective paper improves detection and decoding of QR codes.
- The QR code size should be measured without including silent regions, using the finder pattern as boundaries.
Decoding a QR Code
The decoded QR code string is stored in SpacesARMarker.Data
. The SpacesARMarker
is created every time a QR code is detected, and destroyed when the QR code tracking is lost. Before accessing the SpacesARMarker.Data
value, make sure that SpacesARMarker.IsMarkerDataAvailable
is true since tracking could run before the QR code is decoded.
Tracking a QR Code
Turning on Marker Tracking in the SpacesQRCodeManager
will enable the subsystem to track the pose of any detected QR codes. Turn it off in order to increase performance when not in use.
Enabling and Disabling the SpacesQrCodeManager
Enabling and disabling the SpacesQrCodeManager
component will Start and Stop the subsystem respectively. A restart of the subsystem will be required if there are any modifications for the SpacesQrCodeManager
variables. To restart simply disable and then re-enable the SpacesQrCodeManager
component.
Enabling or disabling Marker Tracking
does not need a subsystem restart to take effect.
SpacesQrCodeManager manager;
manager.enabled = false;
manager.markerSize = new Vector2(20.0f, 20.0f);
manager.markerTrackingMode = MarkerTrackingMode.Dynamic;
manager.enabled = true;
Known Issues
Unstable duplicate code
QR Code Tracking may start tracking an unstable duplicate QR code after several minutes of tracking, especially when the QR Code is located on the outer edge of the camera's field of view.